DSA Tutorial
Linked lists, stacks & queues, hash tables, shortest path, minimum spanning tree, maximum flow, time complexity, dsa reference, dsa examples, dsa the traveling salesman problem.
The Traveling Salesman Problem
The Traveling Salesman Problem states that you are a salesperson and you must visit a number of cities or towns.
Rules : Visit every city only once, then return back to the city you started in.
Goal : Find the shortest possible route.
Except for the Held-Karp algorithm (which is quite advanced and time consuming, (\(O(2^n n^2)\)), and will not be described here), there is no other way to find the shortest route than to check all possible routes.
This means that the time complexity for solving this problem is \(O(n!)\), which means 720 routes needs to be checked for 6 cities, 40,320 routes must be checked for 8 cities, and if you have 10 cities to visit, more than 3.6 million routes must be checked!
Note: "!", or "factorial", is a mathematical operation used in combinatorics to find out how many possible ways something can be done. If there are 4 cities, each city is connected to every other city, and we must visit every city exactly once, there are \(4!= 4 \cdot 3 \cdot 2 \cdot 1 = 24\) different routes we can take to visit those cities.
The Traveling Salesman Problem (TSP) is a problem that is interesting to study because it is very practical, but so time consuming to solve, that it becomes nearly impossible to find the shortest route, even in a graph with just 20-30 vertices.
If we had an effective algorithm for solving The Traveling Salesman Problem, the consequences would be very big in many sectors, like for example chip design, vehicle routing, telecommunications, and urban planning.

Checking All Routes to Solve The Traveling Salesman Problem
To find the optimal solution to The Traveling Salesman Problem, we will check all possible routes, and every time we find a shorter route, we will store it, so that in the end we will have the shortest route.
Good: Finds the overall shortest route.
Bad: Requires an awful lot of calculation, especially for a large amount of cities, which means it is very time consuming.
How it works:
- Check the length of every possible route, one route at a time.
- Is the current route shorter than the shortest route found so far? If so, store the new shortest route.
- After checking all routes, the stored route is the shortest one.
Such a way of finding the solution to a problem is called brute force .
Brute force is not really an algorithm, it just means finding the solution by checking all possibilities, usually because of a lack of a better way to do it.
Speed: {{ inpVal }}
Finding the shortest route in The Traveling Salesman Problem by checking all routes (brute force).
Progress: {{progress}}%
n = {{vertices}} cities
{{vertices}}!={{posRoutes}} possible routes
Show every route: {{showCompares}}
The reason why the brute force approach of finding the shortest route (as shown above) is so time consuming is that we are checking all routes, and the number of possible routes increases really fast when the number of cities increases.
Finding the optimal solution to the Traveling Salesman Problem by checking all possible routes (brute force):
Using A Greedy Algorithm to Solve The Traveling Salesman Problem
Since checking every possible route to solve the Traveling Salesman Problem (like we did above) is so incredibly time consuming, we can instead find a short route by just going to the nearest unvisited city in each step, which is much faster.
Good: Finds a solution to the Traveling Salesman Problem much faster than by checking all routes.
Bad: Does not find the overall shortest route, it just finds a route that is much shorter than an average random route.
- Visit every city.
- The next city to visit is always the nearest of the unvisited cities from the city you are currently in.
- After visiting all cities, go back to the city you started in.
This way of finding an approximation to the shortest route in the Traveling Salesman Problem, by just going to the nearest unvisited city in each step, is called a greedy algorithm .
Finding an approximation to the shortest route in The Traveling Salesman Problem by always going to the nearest unvisited neighbor (greedy algorithm).
As you can see by running this simulation a few times, the routes that are found are not completely unreasonable. Except for a few times when the lines cross perhaps, especially towards the end of the algorithm, the resulting route is a lot shorter than we would get by choosing the next city at random.
Finding a near-optimal solution to the Traveling Salesman Problem using the nearest-neighbor algorithm (greedy):
Other Algorithms That Find Near-Optimal Solutions to The Traveling Salesman Problem
In addition to using a greedy algorithm to solve the Traveling Salesman Problem, there are also other algorithms that can find approximations to the shortest route.
These algorithms are popular because they are much more effective than to actually check all possible solutions, but as with the greedy algorithm above, they do not find the overall shortest route.
Algorithms used to find a near-optimal solution to the Traveling Salesman Problem include:
- 2-opt Heuristic: An algorithm that improves the solution step-by-step, in each step removing two edges and reconnecting the two paths in a different way to reduce the total path length.
- Genetic Algorithm: This is a type of algorithm inspired by the process of natural selection and use techniques such as selection, mutation, and crossover to evolve solutions to problems, including the TSP.
- Simulated Annealing: This method is inspired by the process of annealing in metallurgy. It involves heating and then slowly cooling a material to decrease defects. In the context of TSP, it's used to find a near-optimal solution by exploring the solution space in a way that allows for occasional moves to worse solutions, which helps to avoid getting stuck in local minima.
- Ant Colony Optimization: This algorithm is inspired by the behavior of ants in finding paths from the colony to food sources. It's a more complex probabilistic technique for solving computational problems which can be mapped to finding good paths through graphs.
Time Complexity for Solving The Traveling Salesman Problem
To get a near-optimal solution fast, we can use a greedy algorithm that just goes to the nearest unvisited city in each step, like in the second simulation on this page.
Solving The Traveling Salesman Problem in a greedy way like that, means that at each step, the distances from the current city to all other unvisited cities are compared, and that gives us a time complexity of \(O(n^2) \).
But finding the shortest route of them all requires a lot more operations, and the time complexity for that is \(O(n!)\), like mentioned earlier, which means that for 4 cities, there are 4! possible routes, which is the same as \(4 \cdot 3 \cdot 2 \cdot 1 = 24\). And for just 12 cities for example, there are \(12! = 12 \cdot 11 \cdot 10 \cdot \; ... \; \cdot 2 \cdot 1 = 479,001,600\) possible routes!
See the time complexity for the greedy algorithm \(O(n^2)\), versus the time complexity for finding the shortest route by comparing all routes \(O(n!)\), in the image below.

But there are two things we can do to reduce the number of routes we need to check.
In the Traveling Salesman Problem, the route starts and ends in the same place, which makes a cycle. This means that the length of the shortest route will be the same no matter which city we start in. That is why we have chosen a fixed city to start in for the simulation above, and that reduces the number of possible routes from \(n!\) to \((n-1)!\).
Also, because these routes go in cycles, a route has the same distance if we go in one direction or the other, so we actually just need to check the distance of half of the routes, because the other half will just be the same routes in the opposite direction, so the number of routes we need to check is actually \( \frac{(n-1)!}{2}\).
But even if we can reduce the number of routes we need to check to \( \frac{(n-1)!}{2}\), the time complexity is still \( O(n!)\), because for very big \(n\), reducing \(n\) by one and dividing by 2 does not make a significant change in how the time complexity grows when \(n\) is increased.
To better understand how time complexity works, go to this page .
Actual Traveling Salesman Problems Are More Complex
The edge weight in a graph in this context of The Traveling Salesman Problem tells us how hard it is to go from one point to another, and it is the total edge weight of a route we want to minimize.
So far on this page, the edge weight has been the distance in a straight line between two points. And that makes it much easier to explain the Traveling Salesman Problem, and to display it.
But in the real world there are many other things that affects the edge weight:
- Obstacles: When moving from one place to another, we normally try to avoid obstacles like trees, rivers, houses for example. This means it is longer and takes more time to go from A to B, and the edge weight value needs to be increased to factor that in, because it is not a straight line anymore.
- Transportation Networks: We usually follow a road or use public transport systems when traveling, and that also affects how hard it is to go (or send a package) from one place to another.
- Traffic Conditions: Travel congestion also affects the travel time, so that should also be reflected in the edge weight value.
- Legal and Political Boundaries: Crossing border for example, might make one route harder to choose than another, which means the shortest straight line route might be slower, or more costly.
- Economic Factors: Using fuel, using the time of employees, maintaining vehicles, all these things cost money and should also be factored into the edge weights.
As you can see, just using the straight line distances as the edge weights, might be too simple compared to the real problem. And solving the Traveling Salesman Problem for such a simplified problem model would probably give us a solution that is not optimal in a practical sense.
It is not easy to visualize a Traveling Salesman Problem when the edge length is not just the straight line distance between two points anymore, but the computer handles that very well.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Solving Travelling Salesman Problem in JavaScript
The Travelling Salesman Problem: A Brief Overview
The TSP is an optimization problem, where a salesman is given a list of cities and must determine the shortest possible route that allows him to visit each city once and return to his original location.
Implementing TSP in JavaScript
We will be using a technique called "backtracking" to solve the TSP. Backtracking is a systematic way of trying out different sequence options to find the solution for a problem.
The code provided does not make use of any external library. It's written in pure JavaScript, which means you don't have to import any additional libraries.
Initialize Variables
- shortestDistance = Infinity; : Here, Infinity is a special keyword in JavaScript that represents a value greater than any other number. The shortestDistance variable is used to store the distance of the shortest path found so far during the backtracking process. Initially it is set to Infinity since we are trying to minimize this value as we try out different path sequences.
- shortestPath = []; : This could be used to hold the order of cities that comprise the shortest route. However, it appears some parts of the code might be missing as this variable isn't used in the provided script.
Function Parameters
- graph : This argument should be a two-dimensional array, representing an adjacency matrix of the cities (i.e., graph vertices). Each entry graph[i][j] denotes the distance from city i to city j.
- v : This is an array of boolean values that keep track of which cities (vertices) have been visited. It should have size equal to n .
- currPos : This is a number that represents the current city or position.
- n : It will be a number representing total number of cities.
- count : This counts how many cities have been visited so far.
- cost : Represents cost incurred so far (in term of distances between visited cities).
Running Code
To run your code, you need Node.js installed on your system. Create a file with a .js extension, copy this code into that file and you can execute it using 'node filename.js' on your terminal.
The provided code only determines the minimum distance for TSP; there's no implementation to actually track the order of cities in the shortest path (The shortestPath [] variable isn't used). It's a basic brute-force approach using backtracking, so it might not be efficient for large number of cities. More sophisticated methods might be required for solving larger instances of TSP in a reasonable amount of time.
Running the TSP JavaScript Code
Once you have your code ready, you can run it using Node.js. If you don't have Node.js installed, you can download it from the official website. Then, run the following command in the terminal:
Remember, if you're looking to hire JavaScript developers , understanding these types of problem-solving strategies can be incredibly beneficial.
By following this tutorial, you should now have a working JavaScript solution for the Travelling Salesman Problem. Happy coding!
If you're interested in enhancing this article or becoming a contributing author, we'd love to hear from you.
Please contact Sasha at [email protected] to discuss the opportunity further or to inquire about adding a direct link to your resource. We welcome your collaboration and contributions!
Backtracking
Backtracking is a general algorithm for finding all solutions to some problems, particularly constraint satisfaction problems. It incrementally builds candidates to the solutions and abandons a candidate ('backtracks') as soon as it determines that the candidate cannot be extended to a valid solution. Backtracking is essentially a depth-first search algorithm with pruning. Examples of problems that can be solved using backtracking include the N-Queens problem, Sudoku, and the traveling salesman problem. Learn more about Backtracking from Wikipedia .
Node.js is an open-source, cross-platform, JavaScript runtime environment that executes JavaScript code outside a web browser. It allows developers to use JavaScript to write server-side scripts and build scalable network applications, such as web servers and APIs. Node.js is built on the V8 JavaScript engine from Google Chrome and provides an event-driven, non-blocking I/O model that makes it lightweight and efficient.
Travelling Salesman Problem (TSP)
The Travelling Salesman Problem (TSP) is a classic algorithmic problem in the fields of computer science and operations research. It focuses on optimization and involves a salesman who is given a list of cities and must determine the shortest possible route that allows him to visit each city once and return to his original location. This problem has been fundamental in the study of complex algorithms and has been applied in various fields, including logistics, genetics, and manufacturing.
Maximize Your Productivity with Remote Database & JavaScript Developers Skilled in Rollbar
Elevate Your Engineering Capacity with Remote Database & JavaScript Developers Skilled in SignalR
Maximize Your Team's Potential with Remote Database & JavaScript Developers Skilled in Maven

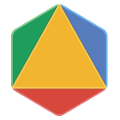
- Google OR-Tools
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Traveling Salesperson Problem
This section presents an example that shows how to solve the Traveling Salesperson Problem (TSP) for the locations shown on the map below.
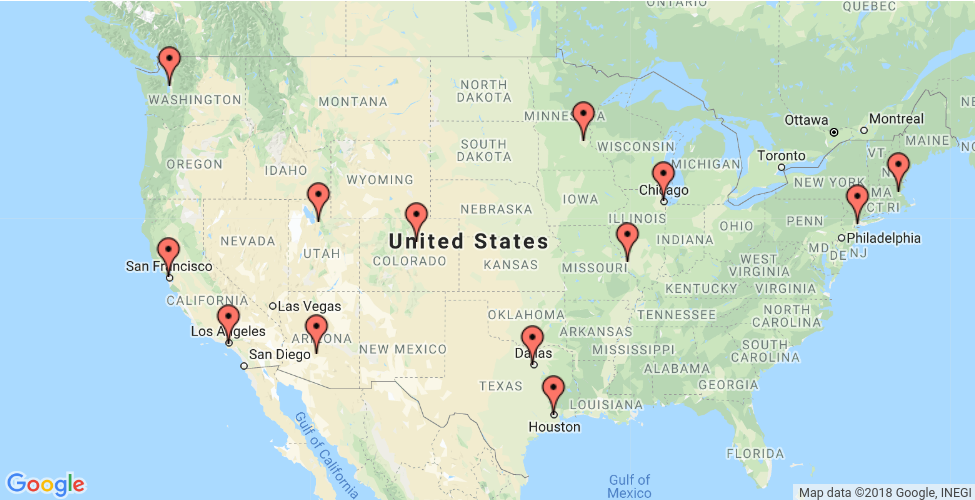
The following sections present programs in Python, C++, Java, and C# that solve the TSP using OR-Tools
Create the data
The code below creates the data for the problem.
The distance matrix is an array whose i , j entry is the distance from location i to location j in miles, where the array indices correspond to the locations in the following order:
The data also includes:
- The number of vehicles in the problem, which is 1 because this is a TSP. (For a vehicle routing problem (VRP), the number of vehicles can be greater than 1.)
- The depot : the start and end location for the route. In this case, the depot is 0, which corresponds to New York.
Other ways to create the distance matrix
In this example, the distance matrix is explicitly defined in the program. It's also possible to use a function to calculate distances between locations: for example, the Euclidean formula for the distance between points in the plane. However, it's still more efficient to pre-compute all the distances between locations and store them in a matrix, rather than compute them at run time. See Example: drilling a circuit board for an example that creates the distance matrix this way.
Another alternative is to use the Google Maps Distance Matrix API to dynamically create a distance (or travel time) matrix for a routing problem.
Create the routing model
The following code in the main section of the programs creates the index manager ( manager ) and the routing model ( routing ). The method manager.IndexToNode converts the solver's internal indices (which you can safely ignore) to the numbers for locations. Location numbers correspond to the indices for the distance matrix.
The inputs to RoutingIndexManager are:
- The number of rows of the distance matrix, which is the number of locations (including the depot).
- The number of vehicles in the problem.
- The node corresponding to the depot.
Create the distance callback
To use the routing solver, you need to create a distance (or transit) callback : a function that takes any pair of locations and returns the distance between them. The easiest way to do this is using the distance matrix.
The following function creates the callback and registers it with the solver as transit_callback_index .
The callback accepts two indices, from_index and to_index , and returns the corresponding entry of the distance matrix.
Set the cost of travel
The arc cost evaluator tells the solver how to calculate the cost of travel between any two locations — in other words, the cost of the edge (or arc) joining them in the graph for the problem. The following code sets the arc cost evaluator.
In this example, the arc cost evaluator is the transit_callback_index , which is the solver's internal reference to the distance callback. This means that the cost of travel between any two locations is just the distance between them. However, in general the costs can involve other factors as well.
You can also define multiple arc cost evaluators that depend on which vehicle is traveling between locations, using the method routing.SetArcCostEvaluatorOfVehicle() . For example, if the vehicles have different speeds, you could define the cost of travel between locations to be the distance divided by the vehicle's speed — in other words, the travel time.
Set search parameters
The following code sets the default search parameters and a heuristic method for finding the first solution:
The code sets the first solution strategy to PATH_CHEAPEST_ARC , which creates an initial route for the solver by repeatedly adding edges with the least weight that don't lead to a previously visited node (other than the depot). For other options, see First solution strategy .
Add the solution printer
The function that displays the solution returned by the solver is shown below. The function extracts the route from the solution and prints it to the console.
The function displays the optimal route and its distance, which is given by ObjectiveValue() .
Solve and print the solution
Finally, you can call the solver and print the solution:
This returns the solution and displays the optimal route.
Run the programs
When you run the programs, they display the following output.
In this example, there's only one route because it's a TSP. But in more general vehicle routing problems, the solution contains multiple routes.
Save routes to a list or array
As an alternative to printing the solution directly, you can save the route (or routes, for a VRP) to a list or array. This has the advantage of making the routes available in case you want to do something with them later. For example, you could run the program several times with different parameters and save the routes in the returned solutions to a file for comparison.
The following functions save the routes in the solution to any VRP (possibly with multiple vehicles) as a list (Python) or an array (C++).
You can use these functions to get the routes in any of the VRP examples in the Routing section.
The following code displays the routes.
For the current example, this code returns the following route:
As an exercise, modify the code above to format the output the same way as the solution printer for the program.
Complete programs
The complete TSP programs are shown below.
Example: drilling a circuit board
The next example involves drilling holes in a circuit board with an automated drill. The problem is to find the shortest route for the drill to take on the board in order to drill all of the required holes. The example is taken from TSPLIB, a library of TSP problems.
Here's scatter chart of the locations for the holes:
The following sections present programs that find a good solution to the circuit board problem, using the solver's default search parameters. After that, we'll show how to find a better solution by changing the search strategy .
The data for the problem consist of 280 points in the plane, shown in the scatter chart above. The program creates the data in an array of ordered pairs corresponding to the points in the plane, as shown below.
Compute the distance matrix
The function below computes the Euclidean distance between any two points in the data and stores it in an array. Because the routing solver works over the integers, the function rounds the computed distances to integers. Rounding doesn't affect the solution in this example, but might in other cases. See Scaling the distance matrix for a way to avoid possible rounding issues.
Add the distance callback
The code that creates the distance callback is almost the same as in the previous example. However, in this case the program calls the function that computes the distance matrix before adding the callback.
Solution printer
The following function prints the solution to the console. To keep the output more compact, the function displays just the indices of the locations in the route.
Main function
The main function is essentially the same as the one in the previous example , but also includes a call to the function that creates the distance matrix.
Running the program
The complete programs are shown in the next section . When you run the program, it displays the following route:
Here's a graph of the corresponding route:
The OR-Tools library finds the above tour very quickly: in less than a second on a typical computer. The total length of the above tour is 2790.
Here are the complete programs for the circuit board example.
Changing the search strategy
The routing solver does not always return the optimal solution to a TSP, because routing problems are computationally intractable. For instance, the solution returned in the previous example is not the optimal route.
To find a better solution, you can use a more advanced search strategy, called guided local search , which enables the solver to escape a local minimum — a solution that is shorter than all nearby routes, but which is not the global minimum. After moving away from the local minimum, the solver continues the search.
The examples below show how to set a guided local search for the circuit board example.
For other local search strategies, see Local search options .
The examples above also enable logging for the search. While logging isn't required, it can be useful for debugging.
When you run the program after making the changes shown above, you get the following solution, which is shorter than the solution shown in the previous section .
For more search options, see Routing Options .
The best algorithms can now routinely solve TSP instances with tens of thousands of nodes. (The record at the time of writing is the pla85900 instance in TSPLIB, a VLSI application with 85,900 nodes. For certain instances with millions of nodes, solutions have been found guaranteed to be within 1% of an optimal tour.)
Scaling the distance matrix
Since the routing solver works over the integers, if your distance matrix has non-integer entries, you have to round the distances to integers. If some distances are small, rounding can affect the solution.
To avoid any issue with rounding, you can scale the distance matrix: multiply all entries of the matrix by a large number — say 100. This multiplies the length of any route by a factor of 100, but it doesn't change the solution. The advantage is that now when you round the matrix entries, the rounding amount (which is at most 0.5), is very small compared to the distances, so it won't affect the solution significantly.
If you scale the distance matrix, you also need to change the solution printer to divide the scaled route lengths by the scaling factor, so that it displays the unscaled distances of the routes.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-01-16 UTC.
Solving the Traveling Salesman Problem
This is a TSP solver in javascript that uses d3.js for visualization. Click a bunch of spots on the map to make "cities", then click "Run" to run the TSP solver. It will display its first guess, then its final guess. See if it does well. (Note, it minimizes flat x/y Euclidean distances, America is there for effect.) Github Repo
Traveling salesman problem
This web page is a duplicate of https://optimization.mccormick.northwestern.edu/index.php/Traveling_salesman_problems
Author: Jessica Yu (ChE 345 Spring 2014)
Steward: Dajun Yue, Fengqi You
The traveling salesman problem (TSP) is a widely studied combinatorial optimization problem, which, given a set of cities and a cost to travel from one city to another, seeks to identify the tour that will allow a salesman to visit each city only once, starting and ending in the same city, at the minimum cost. 1
- 2.1 Graph Theory
- 2.2 Classifications of the TSP
- 2.3 Variations of the TSP
- 3.1 aTSP ILP Formulation
- 3.2 sTSP ILP Formulation
- 4.1 Exact algorithms
- 4.2.1 Tour construction procedures
- 4.2.2 Tour improvement procedures
- 5 Applications
- 7 References
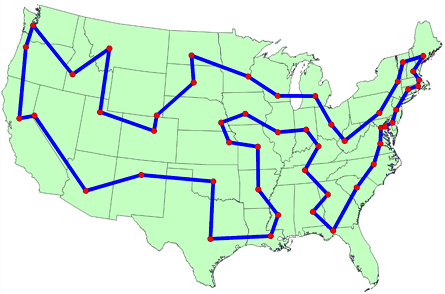
The origins of the traveling salesman problem are obscure; it is mentioned in an 1832 manual for traveling salesman, which included example tours of 45 German cities but gave no mathematical consideration. 2 W. R. Hamilton and Thomas Kirkman devised mathematical formulations of the problem in the 1800s. 2
It is believed that the general form was first studied by Karl Menger in Vienna and Harvard in the 1930s. 2,3
Hassler Whitney, who was working on his Ph.D. research at Harvard when Menger was a visiting lecturer, is believed to have posed the problem of finding the shortest route between the 48 states of the United States during either his 1931-1932 or 1934 seminar talks. 2 There is also uncertainty surrounding the individual who coined the name “traveling salesman problem” for Whitney’s problem. 2
The problem became increasingly popular in the 1950s and 1960s. Notably, George Dantzig, Delber R. Fulkerson, and Selmer M. Johnson at the RAND Corporation in Santa Monica, California solved the 48 state problem by formulating it as a linear programming problem. 2 The methods described in the paper set the foundation for future work in combinatorial optimization, especially highlighting the importance of cutting planes. 2,4
In the early 1970s, the concept of P vs. NP problems created buzz in the theoretical computer science community. In 1972, Richard Karp demonstrated that the Hamiltonian cycle problem was NP-complete, implying that the traveling salesman problem was NP-hard. 4
Increasingly sophisticated codes led to rapid increases in the sizes of the traveling salesman problems solved. Dantzig, Fulkerson, and Johnson had solved a 48 city instance of the problem in 1954. 5 Martin Grötechel more than doubled this 23 years later, solving a 120 city instance in 1977. 5 Enoch Crowder and Manfred W. Padberg again more than doubled this in just 3 years, with a 318 city solution. 5
In 1987, rapid improvements were made, culminating in a 2,392 city solution by Padberg and Giovanni Rinaldi. In the following two decades, David L. Appelgate, Robert E. Bixby, Vasek Chvátal, & William J. Cook led the cutting edge, solving a 7,397 city instance in 1994 up to the current largest solved problem of 24,978 cities in 2004. 5
Description
Graph theory.
In the context of the traveling salesman problem, the verticies correspond to cities and the edges correspond to the path between those cities. When modeled as a complete graph, paths that do not exist between cities can be modeled as edges of very large cost without loss of generality. 6 Minimizing the sum of the costs for Hamiltonian cycle is equivalent to identifying the shortest path in which each city is visiting only once.
Classifications of the TSP
The TRP can be divided into two classes depending on the nature of the cost matrix. 3,6
- Applies when the distance between cities is the same in both directions
- Applies when there are differences in distances (e.g. one-way streets)
An ATSP can be formulated as an STSP by doubling the number of nodes. 6
Variations of the TSP
Formulation
The objective function is then given by
To ensure that the result is a valid tour, several contraints must be added. 1,3
There are several other formulations for the subtour elimnation contraint, including circuit packing contraints, MTZ constraints, and network flow constraints.
aTSP ILP Formulation
The integer linear programming formulation for an aTSP is given by
sTSP ILP Formulation
The symmetric case is a special case of the asymmetric case and the above formulation is valid. 3, 6 The integer linear programming formulation for an sTSP is given by
Exact algorithms
Branch-and-bound algorithms are commonly used to find solutions for TSPs. 7 The ILP is first relaxed and solved as an LP using the Simplex method, then feasibility is regained by enumeration of the integer variables. 7
Other exact solution methods include the cutting plane method and branch-and-cut. 8
Heuristic algorithms
Given that the TSP is an NP-hard problem, heuristic algorithms are commonly used to give a approximate solutions that are good, though not necessarily optimal. The algorithms do not guarantee an optimal solution, but gives near-optimal solutions in reasonable computational time. 3 The Held-Karp lower bound can be calculated and used to judge the performance of a heuristic algorithm. 3
There are two general heuristic classifications 7 :
- Tour construction procedures where a solution is gradually built by adding a new vertex at each step
- Tour improvement procedures where a feasbile solution is improved upon by performing various exchanges
The best methods tend to be composite algorithms that combine these features. 7
Tour construction procedures
Tour improvement procedures
Applications
The importance of the traveling salesman problem is two fold. First its ubiquity as a platform for the study of general methods than can then be applied to a variety of other discrete optimization problems. 5 Second is its diverse range of applications, in fields including mathematics, computer science, genetics, and engineering. 5,6
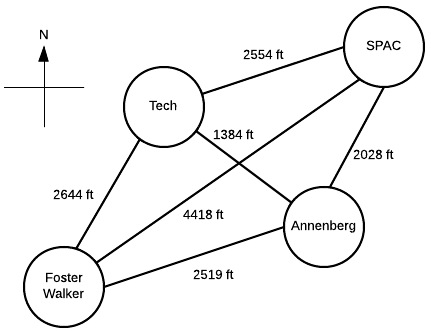
Suppose a Northwestern student, who lives in Foster-Walker , has to accomplish the following tasks:
- Drop off a homework set at Tech
- Work out a SPAC
- Complete a group project at Annenberg
Distances between buildings can be found using Google Maps. Note that there is particularly strong western wind and walking east takes 1.5 times as long.
It is the middle of winter and the student wants to spend the least possible time walking. Determine the path the student should take in order to minimize walking time, starting and ending at Foster-Walker.
Start with the cost matrix (with altered distances taken into account):
Method 1: Complete Enumeration
All possible paths are considered and the path of least cost is the optimal solution. Note that this method is only feasible given the small size of the problem.
From inspection, we see that Path 4 is the shortest. So, the student should walk 2.28 miles in the following order: Foster-Walker → Annenberg → SPAC → Tech → Foster-Walker
Method 2: Nearest neighbor
Starting from Foster-Walker, the next building is simply the closest building that has not yet been visited. With only four nodes, this can be done by inspection:
- Smallest distance is from Foster-Walker is to Annenberg
- Smallest distance from Annenberg is to Tech
- Smallest distance from Tech is to Annenberg ( creates a subtour, therefore skip )
- Next smallest distance from Tech is to Foster-Walker ( creates a subtour, therefore skip )
- Next smallest distance from Tech is to SPAC
- Smallest distance from SPAC is to Annenberg ( creates a subtour, therefore skip )
- Next smallest distance from SPAC is to Tech ( creates a subtour, therefore skip )
- Next smallest distance from SPAC is to Foster-Walker
So, the student would walk 2.54 miles in the following order: Foster-Walker → Annenberg → Tech → SPAC → Foster-Walker
Method 3: Greedy
With this method, the shortest paths that do not create a subtour are selected until a complete tour is created.
- Smallest distance is Annenberg → Tech
- Next smallest is SPAC → Annenberg
- Next smallest is Tech → Annenberg ( creates a subtour, therefore skip )
- Next smallest is Anneberg → Foster-Walker ( creates a subtour, therefore skip )
- Next smallest is SPAC → Tech ( creates a subtour, therefore skip )
- Next smallest is Tech → Foster-Walker
- Next smallest is Annenberg → SPAC ( creates a subtour, therefore skip )
- Next smallest is Foster-Walker → Annenberg ( creates a subtour, therefore skip )
- Next smallest is Tech → SPAC ( creates a subtour, therefore skip )
- Next smallest is Foster-Walker → Tech ( creates a subtour, therefore skip )
- Next smallest is SPAC → Foster-Walker ( creates a subtour, therefore skip )
- Next smallest is Foster-Walker → SPAC
So, the student would walk 2.40 miles in the following order: Foster-Walker → SPAC → Annenberg → Tech → Foster-Walker
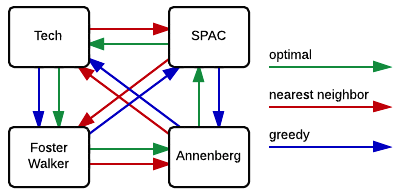
As we can see in the figure to the right, the heuristic methods did not give the optimal solution. That is not to say that heuristics can never give the optimal solution, just that it is not guaranteed.
Both the optimal and the nearest neighbor algorithms suggest that Annenberg is the optimal first building to visit. However, the optimal solution then goes to SPAC, while both heuristic methods suggest Tech. This is in part due to the large cost of SPAC → Foster-Walker. The heuristic algorithms cannot take this future cost into account, and therefore fall into that local optimum.
We note that the nearest neighbor and greedy algorithms give solutions that are 11.4% and 5.3%, respectively, above the optimal solution. In the scale of this problem, this corresponds to fractions of a mile. We also note that neither heuristic gave the worst case result, Foster-Walker → SPAC → Tech → Annenberg → Foster-Walker.
Only tour building heuristics were used. Combined with a tour improvement algorithm (such as 2-opt or simulated annealing), we imagine that we may be able to locate solutions that are closer to the optimum.
The exact algorithm used was complete enumeration, but we note that this is impractical even for 7 nodes (6! or 720 different possibilities). Commonly, the problem would be formulated and solved as an ILP to obtain exact solutions.
- Vanderbei, R. J. (2001). Linear programming: Foundations and extensions (2nd ed.). Boston: Kluwer Academic.
- Schrijver, A. (n.d.). On the history of combinatorial optimization (till 1960).
- Matai, R., Singh, S., & Lal, M. (2010). Traveling salesman problem: An overview of applications, formulations, and solution approaches. In D. Davendra (Ed.), Traveling Salesman Problem, Theory and Applications . InTech.
- Junger, M., Liebling, T., Naddef, D., Nemhauser, G., Pulleyblank, W., Reinelt, G., Rinaldi, G., & Wolsey, L. (Eds.). (2009). 50 years of integer programming, 1958-2008: The early years and state-of-the-art surveys . Heidelberg: Springer.
- Cook, W. (2007). History of the TSP. The Traveling Salesman Problem . Retrieved from http://www.math.uwaterloo.ca/tsp/history/index.htm
- Punnen, A. P. (2002). The traveling salesman problem: Applications, formulations and variations. In G. Gutin & A. P. Punnen (Eds.), The Traveling Salesman Problem and its Variations . Netherlands: Kluwer Academic Publishers.
- Laporte, G. (1992). The traveling salesman problem: An overview of exact and approximate algorithms. European Journal of Operational Research, 59 (2), 231–247.
- Goyal, S. (n.d.). A suvey on travlling salesman problem.
Navigation menu
Search This Blog
Travelling salesman problem in javascript, posted by om prakash, you may like these posts, post a comment, popular posts.
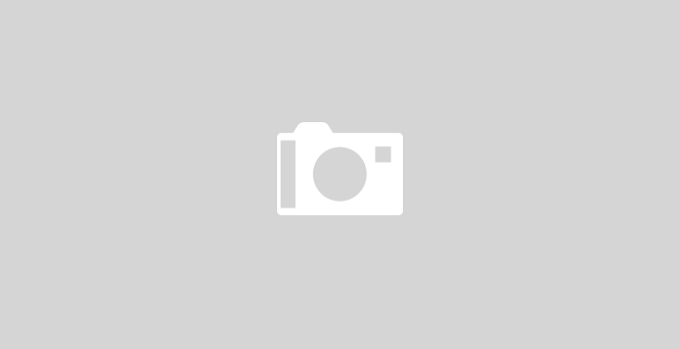
luck balance hacker rank solution javascript
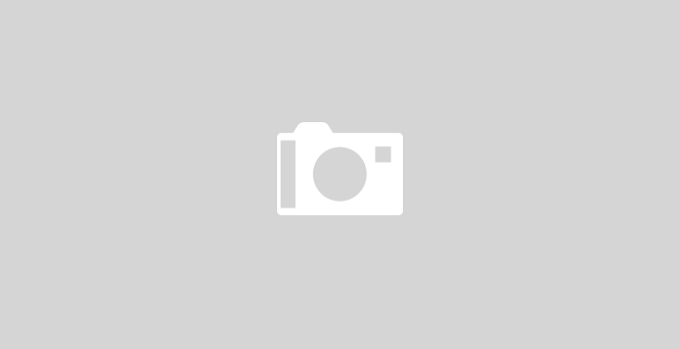
next greater element I leetcode solution
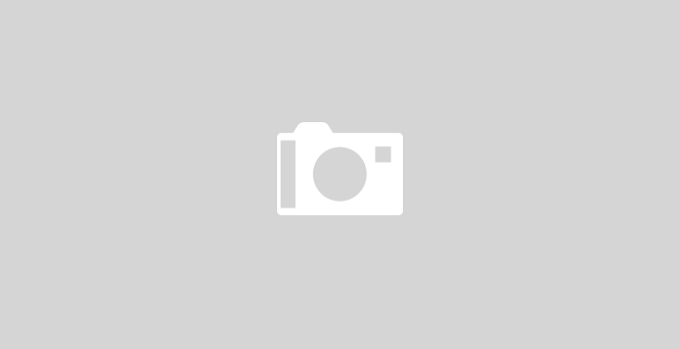
marc's cakewalk hacker rank solution javascript
- hackerrank 151
- data structure 150
- backtracking 25
- linked list 22
- binary search tree 11
- dynamic programming 11
- expression 6
- javascript 6
- bst operations 4
- dynamic progammming 2
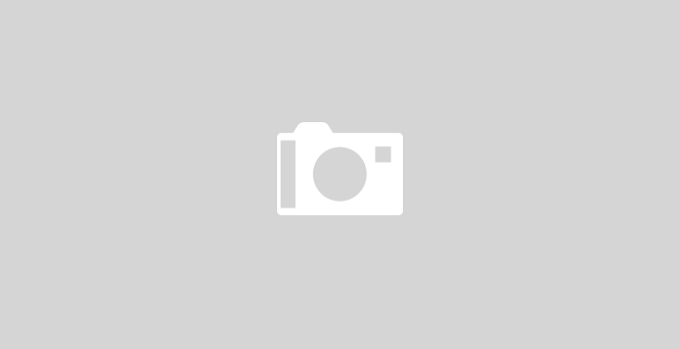
grid challenge hacker rank solution javascript
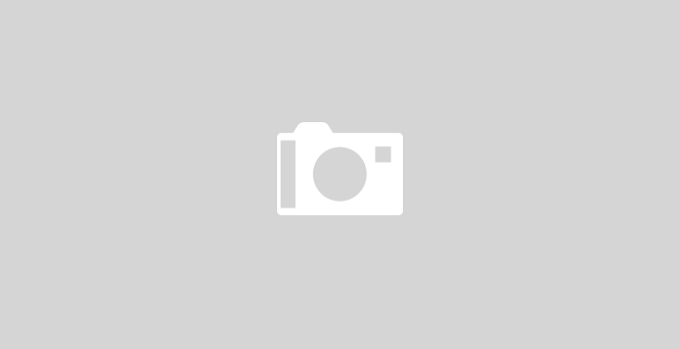
minimum absolute difference in an array hacker rank solution javascript
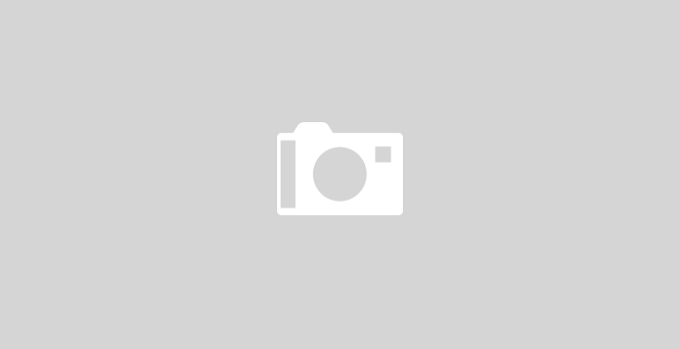
anagram hacker rank solution javascript
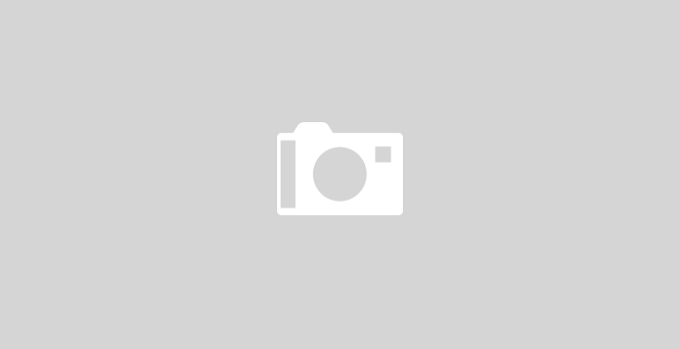
lily's homework hackerrank solution in javascript
Contact form.
Travelling Salesman Problem : Easiest Approach to Implement using Dynamic Programming
Nov 28, 2017
Travelling Salesman Problem with Code
Given a set of cities(nodes), find a minimum weight Hamiltonian Cycle/Tour.
Concepts Used :
Graphs, Bitmasking, Dynamic Programming
Run on IDE ‘O(2^n * n^2)’
- Machine Learning Basics Webinar | Prateek Narang »
Recent articles
Machine Learning Basics Webinar | Prateek Narang 07 Nov 2017
Javascript Promises 06 Nov 2017
var, let and const in Javascript 01 Nov 2017
- Tutorial (19)
- Webinar (7)
- Workshop (3)
- Hackerblocks (9)
- Interview experience (8)
- Work experiences (3)
- Talks and conferences (4)
- Javascript (8)
- Competitive.coding (1)
Coding Blocks
Try RoadWarrior free for 7 days
Solving the Traveling Salesman Problem
Get home early with RoadWarrior.
Enter your stops, optimize your routes, manage your team – quickly and efficiently.
Imagine a salesman who needs to visit multiple cities, but he wants to minimize the distance traveled and return to the starting point. This classic problem, known as the Traveling Salesman Problem (TSP), has been a subject of study for over a century. The applications of TSP are widespread, from logistics and agriculture to astronomy and computer-generated art. In this blog post, we will delve into the world of the Traveling Salesman Problem, exploring its history, algorithms, and real-world applications.
Short Summary
- The Traveling Salesman Problem is a complex problem of finding an optimal route for a round trip.
- Solutions to the TSP include NP-hard classification, brute force approach, dynamic programming and Christofides’ Algorithm.
- Route planning software such as OptimoRoute provide businesses with efficient tools for tackling the TSP, thereby improving their operations and saving resources.
Understanding the Traveling Salesman Problem
The Traveling Salesman Problem (TSP) is a problem of determining the most efficient route for a round trip, with the objective of maintaining the minimum cost and distance traveled. It serves as a foundational problem to test the limits of efficient computation in theoretical computer science.
The salesman’s objective in the TSP is to find a minimum weight Hamiltonian cycle, which maintains both the travel costs and the distance traveled at a minimum.
Theoretical Background
The TSP is classified as an NP-hard problem. This shows that the number of solution sequences grows rapidly with the number of cities. The brute force approach to resolving the TSP involves examining each round-trip route to ascertain the shortest one. However, as the number of cities increases, the number of round-trips to check can quickly surpass the capability of the most powerful computers. This limitation has led to the development of more sophisticated algorithms to tackle the TSP, such as dynamic programming and approximation algorithms.
The Hamiltonian Cycle Problem, also known as the Hamiltonian cycle problem, inquires whether there exists a closed walk in a graph that visits each vertex precisely once and is closely related to the TSP. Both problems have been studied extensively by computer scientists due to their implications in complexity theory and the P versus NP problem.
Optimal vs. Approximate Solutions
In solving the TSP, there is a distinction between optimal solutions and approximate solutions. Optimal solutions are the most advantageous route, while approximate solutions are round-trip routes whose lengths approach that of the most advantageous route. The brute force approach involves determining every potential solution and subsequently selecting the most advantageous one. However, this method is computationally intractable for large TSP instances.
One notable approximate solution is Christofides’ algorithm, which begins by determining the shortest spanning tree and subsequently converting it into a round-trip route. This algorithm guarantees a response that is, at most, 1.5 times the optimal solution. While not always yielding the optimal solution, approximate algorithms like Christofides’ algorithm provide a more feasible approach to solving the TSP.
Evolution of TSP Algorithms
TSP algorithms have been in existence since the 1950s, when the initial brute force approach was formulated. Subsequently, more sophisticated techniques such as dynamic programming and Christofides’ algorithm have been developed. These advanced algorithms have enabled researchers and practitioners to find near-optimal solutions to the TSP more quickly and efficiently.
By leveraging these algorithms, it is possible to solve the TSP in a fraction of the time.

Brute Force Approach
The brute force approach to TSP involves attempting all potential solutions, making it the most time-consuming and expensive method. As the number of destinations increases, the number of roundtrips likewise increases exponentially, rendering it computationally intractable even for the most powerful computers. Therefore, the brute force approach is not considered to be a viable solution for large TSP instances.
Despite its limitations, the brute force approach serves an important role in the history of TSP algorithms. It represents the initial attempt to solve the TSP and laid the groundwork for the development of more advanced and efficient algorithms.
Dynamic Programming
Dynamic programming is a technique employed to address intricate issues by segmenting them into more manageable subproblems and resolving them one at a time. It is regularly utilized to resolve the Traveling Salesman Problem due to its ability to avoid redundant calculations and identify the shortest route that visits all cities exactly once. The dynamic programming approach is more scalable than the brute force approach, as it can be employed to solve problems of any size.
However, dynamic programming is not without its limitations. It can be computationally expensive and time-consuming, as it necessitates solving a substantial number of subproblems. Despite these drawbacks, dynamic programming remains a popular and effective method for solving the TSP.
Christofides’ Algorithm
Christofides’ algorithm is an algorithm for obtaining approximate solutions to the Traveling Salesman Problem. It involves constructing a minimum spanning tree and then discovering a minimum-weight perfect matching on the odd-degree vertices of the tree. This algorithm, developed in the 1970s, utilizes a combination of graph theory and heuristics to address the TSP.
The significance of Christofides’ algorithm lies in its ability to yield routes that are guaranteed to be no more than 50 percent longer than the shortest route. While it may not always provide the optimal solution, its development marked a substantial improvement in the pursuit of efficient TSP algorithms.
Recent Advances in TSP Algorithms
In recent years, computer scientists have made significant advancements in TSP algorithms. These breakthroughs include:
- Approximation algorithms
- Metaheuristic approaches
- Local search operators
- Anytime Automatic Algorithm Selection
These cutting-edge algorithms have enabled researchers to find even more efficient solutions to the TSP, further demonstrating the importance of continued research and development in this area.
Geometry of Polynomials
Oveis Gharan and Nathan Klein used the geometry of polynomials approach to solve the TSP by representing the problem as a polynomial with variables corresponding to the edges between all the cities. This approach permits the utilization of geometric techniques and algorithms to discover an optimal or approximate solution to the problem, including determining the starting and ending point of the route.
This innovative method showcases the potential for leveraging mathematical techniques and geometrical properties in solving the TSP. As research progresses, it is expected that even more efficient algorithms and approaches will be developed, further pushing the boundaries of our understanding of the TSP.
Fractional Solutions and Rounding Techniques
Amin Saberi and Arash Asadpour developed a general rounding technique that employs randomness in an attempt to select a whole-number solution that preserves as many characteristics of the fractional solution as possible. The use of fractional solutions and rounding techniques can be utilized to construct effective approximation algorithms for the TSP.
Saberi, Gharan, and Singh implemented this general rounding technique to formulate a new approximation algorithm for the TSP. As computer scientists continue to explore new approaches and techniques, it is likely that even more powerful and efficient algorithms will be developed to tackle the complex TSP.
Practical Applications of TSP Solutions
TSP solutions are employed in a variety of industries, including logistics, astronomy, agriculture, and vehicle routing. Its applications range from planning efficient delivery routes and optimizing telescope trajectories to designing microchips and creating computer-generated art.
The widespread use of TSP solutions underscores the importance of continued research and development in this area, as advancements in TSP algorithms have the potential to positively impact numerous sectors.
Route Optimization
Route optimization is the process of determining the most efficient routes for various applications. In logistics, for example, TSP solutions can assist in enhancing efficiency in the last mile. By employing optimization techniques, businesses can reduce the number of stops, minimize total distance traveled, and ultimately save on fuel and labor costs.
The Vehicle Routing Problem (VRP), a generalized form of the TSP, focuses on discovering the most efficient set of routes or paths for multiple vehicles and hundreds of delivery locations. By utilizing TSP solutions and optimization techniques, companies can greatly improve their overall efficiency and productivity, leading to significant cost savings and improved customer service.
Last-Mile Delivery Challenges
The last-mile delivery problem refers to the final stage of a supply chain. It involves transporting goods from a transportation hub, such as a depot or warehouse, to the ultimate recipient. TSP solutions play a crucial role in addressing this challenge by optimizing delivery routes, reducing the number of stops, and minimizing total distance traveled.
For example, the Traveling Salesman Problem with Time Windows (TSPTW) approach considers specific time constraints for each delivery location, ensuring that deliveries are made within a specified time frame. By employing TSP algorithms and optimization techniques, businesses can:
- Overcome last-mile delivery challenges
- Improve overall efficiency
- Achieve cost savings
- Enhance customer satisfaction
TSP Solvers for Real-World Problems
Modern TSP solvers use advanced algorithms to provide near-optimal solutions quickly. These solvers include the routingpy library, real-life TSP and VRP solvers, and state-of-the-art TSP solvers based on local search.
By leveraging these powerful algorithms, businesses and researchers can tackle real-world TSP problems with greater efficiency and accuracy.
Branch and Bound Method
The branch and bound method is an algorithm utilized to address optimization problems, such as the TSP. It functions by exhaustively examining all potential solutions and identifying the most advantageous one. By dividing the problem into smaller subproblems and determining the optimal solution for each subproblem, the branch and bound method permits a more efficient and precise resolution to the problem.
Although the branch and bound method is computationally expensive, it has several advantages, such as being relatively straightforward to implement and capable of addressing large-scale problems. Its application in solving real-life TSP instances showcases its potential in effectively optimizing routes and minimizing costs.
Nearest Neighbor Method
The Nearest Neighbor algorithm is a greedy algorithm that finds the closest unvisited node and adds it to the sequencing until all nodes are included in the tour. While it rarely yields the optimal solution, particularly for large and intricate instances, it can be utilized effectively as a means to generate an initial feasible solution quickly.
This initial solution can then be supplied into a more sophisticated local search algorithm for further optimization. The Nearest Neighbor algorithm demonstrates that even relatively simple algorithms can play a valuable role in providing quick and feasible solutions to real-world TSP problems.
Route Planning Software for TSP
Utilizing route planning software to solve TSP problems in various industries offers numerous benefits. These software solutions, such as Route4Me, leverage advanced algorithms like Dijkstra’s Algorithm to quickly identify the most efficient route for a team.
By implementing route planning software, businesses can improve efficiency, reduce costs, and enhance customer satisfaction.
Advantages of Route Planning Software
Route planning software can help businesses in the following ways:
- Optimize routes
- Decrease the number of stops
- Minimize the total distance traveled
- Increase efficiency and productivity
- Reduce fuel and labor costs by optimizing routes and minimizing the time spent on route planning and decision-making.
Improved customer service is another benefit of route planning software. By optimizing routes and ensuring timely deliveries, businesses can enhance their reputation and build customer loyalty. In an increasingly competitive market, utilizing route planning software can give businesses a critical edge in delivering exceptional service and maintaining customer satisfaction.
Examples of Route Planning Solutions
There are numerous route planning solutions available for addressing the TSP, including vehicle routing software, optimization algorithms, and Excel sheets with order details and addresses. These solutions offer businesses a variety of options to choose from, depending on their specific needs and requirements.
Some examples of popular route planning software include OptimoRoute and Straightaway. These software solutions can help businesses tackle TSP problems efficiently, saving time and resources while improving overall operations. By leveraging advanced algorithms and powerful tools, route planning software provides a valuable solution for businesses looking to optimize their logistics and delivery processes.
Throughout this blog post, we have explored the fascinating world of the Traveling Salesman Problem, delving into its history, algorithms, and practical applications. From the early brute force approach to modern approximation algorithms and route planning software, the TSP continues to challenge and inspire researchers, computer scientists, and businesses alike. As we continue to push the boundaries of our understanding of the TSP, the potential for new and innovative solutions to real-world problems remains vast and exciting.
Frequently Asked Questions
Has anyone solved the traveling salesman problem.
No one has successfully come up with an algorithm to efficiently solve every traveling salesman problem, despite notable progress being made over the years.
What is the Traveling Salesman Problem (TSP)?
The Traveling Salesman Problem is an optimization problem that seeks to determine the most efficient route for a round trip, with the aim of minimizing cost and distance traveled.
What are some examples of industries that benefit from TSP solutions?
TSP solutions are widely used in industries such as logistics, astronomy, agriculture, and vehicle routing, providing a range of benefits.
What is the difference between optimal and approximate solutions in TSP?
Optimal solutions in TSP provide the most advantageous route, while approximate solutions offer a similar route whose length is close to that of the optimal solution.
These solutions can be used to solve a variety of problems, such as finding the shortest route between two cities or the most efficient way to deliver goods. They can also be used to optimize the use of resources, such as time and money.
What is an example of a modern TSP solver?
Routingpy is an example of a modern TSP solver, providing comprehensive tools to address the Traveling Salesman Problem and Vehicle Routing Problem.
It offers a range of features, including an intuitive user interface, fast computation times, and a wide range of optimization algorithms. It also provides a comprehensive set of tools for analyzing and visualizing the results of the optimization process.
Related Articles
Walmart spark delivery: a lucrative opportunity for independent contractors.
11 minute read
Android Route Planning Simplified: The Best Apps on the Market
7 minute read
Best Route Planners for iPhone: Navigating the App Choices
Joe's Blog
What's up in my mind
Grab the RSS feed
Travelling Salesman Problem in JavaScript Canvas
Posted by joe | Filed under Javascript , Software Development
A long a time ago – when I was student at university – I wrote a small, but somewhat impressing program. It is a heuristic (and thus suboptimal) solver for the travelling salesman problem, using an algorithm from the field of neuronal network which is called a self organizing feature map
You can read details on the neuronal network and the algorithm in my university paper self organising maps based on a Kohonen neuronal network (in german) or follow the literature references given.
Now I took the chance of a rainy winter evening to convert the original code to JavaScript and render the output with the HTML5 Canvas 2D API – for me the chance to learn and keep up with the different HTML5 specs which have now step by step have become reality. So first have a look on the application:
Simulation status
If you look at the JavaScript code, the part which takes care of the painting is remarkable simple, consisting of 3 sections. Creating the drawing context on the canvas element: elem = document.getElementById('#canvas'); ctx = elem.getContext('2d'); height = elem.height; width= elem.width; Drawing the cities as filled circles: var centerX = city.x * width + 0.5; var centerY = height - city.y * height + 0.5; ctx.fillStyle = "#C06060"; ctx.beginPath(); ctx.arc(centerX, centerY, 3, 0, Math.PI*2, true); ctx.closePath(); ctx.fill(); and drawing the candidates and connecting them to a tour: ctx.strokeStyle = "#80D080"; ctx.beginPath(); // move to first node n = neurons.start; ctx.moveTo(n.x * width + 0.5, height - n.y * height + 0.5);
for each node n // each node as a small dot var centerX = n.x * width + 0.5; var centerY = height - n.y * height + 0.5; ctx.fillStyle = "#208020"; ctx.fillRect(centerX, centerY, 1, 1); // draw a line to the next node ctx.lineTo(n.right.x * width + 0.5, height - n.right.y * height + 0.5);
// draw all lines ctx.lineWidth = 1; ctx.stroke(); ctx.closePath();
You can checkout the source code here: https://github.com/kifj/tsp-js
Tagged : HTML5 Canvas , Javascript , Kohonen Network , Neuronal Network , Traveling Salesman Problem
Comments (14) | March 10th, 2013
14 Responses to “Travelling Salesman Problem in JavaScript Canvas”
Johanes, this is a great piece of code. I’m really impressed. The algorithm is damn effective. All those clove hitches (aka sub optimalities) can be solved via “moving window” of 3 , 4 or 5 isolated vertices. Even with this improvement (coz it is finite) the code would be extremely fast as #nodes grows.
All my regards, Alex
[…] of Traveling Salesman Problem or TSP. there are various ways to address it, often involving neural networks or genetic algorithm (I believe the latter is more robust) but there’s another approach […]
I’m really impress by the effectivness of you algorithm. I have been looking for a javascrip implementation for a long time.
It’s indeed suboptimal, but as metioned by Alex it can be solved quite easily .
Thanks for your contribution.
Best regards, Pierre
Hello. I’m new to all this, but I want to learn. Why exactly is it suboptimal? And what would make it optimal? I didn’t understand the “moving windows” reference, nor am I familiar with neural networks or genetic algorithms although I am about to research them right now.
Any help in understanding would be greatly appreciated.
Hi Joe, I came across your blog when I was searching for TSP implementation in javascript. I am making a project for my college. Can you please mail me the javascript code to the mail specified above.
Thanks for the help
basically everything is on the web page, but hard to find in all that WordPress code. I sent you a mail, with the HTML pieces. The JavaScript is linked and you can download the file.
Great job. Thanks again for sharing.
I am also working on a project linked to Traveling Salesman Problem.
May I ask you to send me the javascript code as well?
Thanks in advance
I got very intrigued by your solution after reading your blog. If you do not mind to send me your solution as well.
Also, just curious, why not to share your solution through GitHub or other code sharing portal so that people in academia could benefit from it?
Convinced, never thought there is much interest in that
https://github.com/kifj/tsp-js
Thanks so much for sharing this, it’s really impressive. I am very curious about the gain and alpha parameters used by the algorithm. Would you please provide a brief, layman’s explanation of what those parameters do and how they might affect the result? Or if there are any recommended guidelines for choosing them? Thanks!!!
First of all, I didn’t invent the algorithm and its parameters. If you are really interested in the theory and explanation, I would recommend the original paper describing the details.
[AVT88] Bernhard Angeniol, Gael De La Croix Vaubois & Jean-Yves Le Texier Self-Organizing Feature Maps and the Travelling Salesman Problem, Neural Networks, Vol. 1, pp. 289-293, 1988
The short explanation for G and alpha: high values of G causes neighbours to be moved with each node. alpha describes the reduction of G after each round – the learning rate. Both parameters influence the convergence speed of the algorrithm (and by that the qualitiy of the solution). For a given TSP I would expect that you can iterate from faster to slower convergence(starting with high G and alpha and reducing it), until the algorithms is too slow or the solution is not improving enough. The speed and quality is heavily influenced of the TSP you want to solve (cluster vs. true random cities). So these parameters are empirically determined.
Thanks for sharing this. This is one of the fastest js implemtations Ive seen. would it be posible to send the html pieces and link as Abhijeet Bhattacharya asked for to my included eail address. Your right
thank you so much, this is excellent
Many thanks for sharing this great code. I have a problem case same as above but need to code in SQL Server. I am not good in the algorithm of Nueron Network. I am very appreciated if you contract me to help me.
Hope to get contact from you
Thanks to Fil, he pointed me to https://observablehq.com/@fil/som-tsp for his version of this, and this here https://diego.codes/post/som-tsp/ with a very good explanation in text and a python version.
Leave a Reply
Name (required)
Mail (will not be published) (required)
XHTML: You can use these tags: <a href="" title=""> <abbr title=""> <acronym title=""> <b> <blockquote cite=""> <cite> <code> <del datetime=""> <em> <i> <q cite=""> <s> <strike> <strong>
Your Message
- Android (1)
- Books (105)
- HikingTracks (46)
- Javascript (2)
- JBoss AS7 (6)
- Rasbperry Pi (1)
- Software Development (16)
- Vacation (6)
Contact Info
Phone: +49 160 7276508
Address: Schillerstr. 32, 76135 Karlsruhe, Germany
E-mail: [email protected]
- Add to Google
- Google+ Profile
Contributors
- X1 HikingTracks

© 2024 Joe's Blog
CSS | XHTML | Home | Back to Top
- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Dynamic Programming or DP
- What is memoization? A Complete tutorial
- Dynamic Programming (DP) Tutorial with Problems
- Tabulation vs Memoization
- Optimal Substructure Property in Dynamic Programming | DP-2
- Overlapping Subproblems Property in Dynamic Programming | DP-1
- Steps for how to solve a Dynamic Programming Problem
Advanced Topics
- Bitmasking and Dynamic Programming | Set 1 (Count ways to assign unique cap to every person)
- Digit DP | Introduction
- Sum over Subsets | Dynamic Programming
Easy problems in Dynamic programming
- Count all combinations of coins to make a given value sum (Coin Change II)
- Subset Sum Problem
- Introduction and Dynamic Programming solution to compute nCr%p
- Cutting a Rod | DP-13
- Painting Fence Algorithm
- Longest Common Subsequence (LCS)
- Longest Increasing Subsequence (LIS)
- Longest subsequence such that difference between adjacents is one
- Maximum size square sub-matrix with all 1s
- Min Cost Path | DP-6
- Longest Common Substring (Space optimized DP solution)
- Count ways to reach the nth stair using step 1, 2 or 3
- Count Unique Paths in matrix
- Unique paths in a Grid with Obstacles
Medium problems on Dynamic programming
- 0/1 Knapsack Problem
- Printing Items in 0/1 Knapsack
- Unbounded Knapsack (Repetition of items allowed)
- Egg Dropping Puzzle | DP-11
- Word Break Problem | DP-32
- Vertex Cover Problem (Dynamic Programming Solution for Tree)
- Tile Stacking Problem
- Box Stacking Problem | DP-22
- Partition problem | DP-18
Travelling Salesman Problem using Dynamic Programming
- Longest Palindromic Subsequence (LPS)
- Longest Common Increasing Subsequence (LCS + LIS)
- Find all distinct subset (or subsequence) sums of an array
- Weighted Job Scheduling
- Count Derangements (Permutation such that no element appears in its original position)
- Minimum insertions to form a palindrome | DP-28
- Ways to arrange Balls such that adjacent balls are of different types
Hard problems on Dynamic programming
- Palindrome Partitioning
- Word Wrap Problem
- The Painter's Partition Problem
- Program for Bridge and Torch problem
- Matrix Chain Multiplication | DP-8
- Printing brackets in Matrix Chain Multiplication Problem
- Maximum sum rectangle in a 2D matrix | DP-27
- Maximum profit by buying and selling a share at most k times
- Minimum cost to sort strings using reversal operations of different costs
- Count of AP (Arithmetic Progression) Subsequences in an array
- Introduction to Dynamic Programming on Trees
- Maximum height of Tree when any Node can be considered as Root
- Longest repeating and non-overlapping substring
- Top 20 Dynamic Programming Interview Questions
Travelling Salesman Problem (TSP):
Given a set of cities and the distance between every pair of cities, the problem is to find the shortest possible route that visits every city exactly once and returns to the starting point. Note the difference between Hamiltonian Cycle and TSP. The Hamiltonian cycle problem is to find if there exists a tour that visits every city exactly once. Here we know that Hamiltonian Tour exists (because the graph is complete) and in fact, many such tours exist, the problem is to find a minimum weight Hamiltonian Cycle.
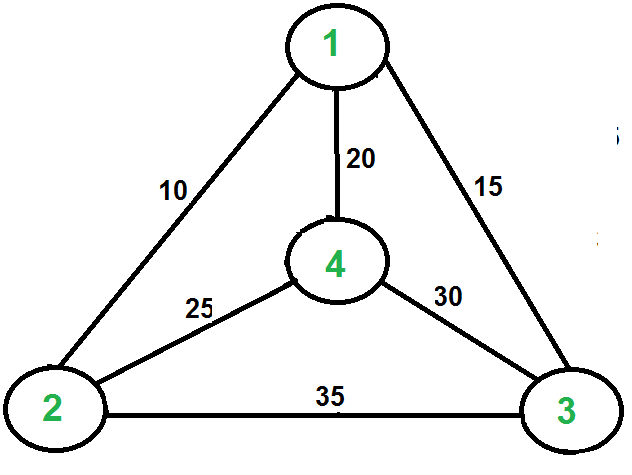
For example, consider the graph shown in the figure on the right side. A TSP tour in the graph is 1-2-4-3-1. The cost of the tour is 10+25+30+15 which is 80. The problem is a famous NP-hard problem. There is no polynomial-time know solution for this problem. The following are different solutions for the traveling salesman problem.
Naive Solution:
1) Consider city 1 as the starting and ending point.
2) Generate all (n-1)! Permutations of cities.
3) Calculate the cost of every permutation and keep track of the minimum cost permutation.
4) Return the permutation with minimum cost.
Time Complexity: ?(n!)
Dynamic Programming:
Let the given set of vertices be {1, 2, 3, 4,….n}. Let us consider 1 as starting and ending point of output. For every other vertex I (other than 1), we find the minimum cost path with 1 as the starting point, I as the ending point, and all vertices appearing exactly once. Let the cost of this path cost (i), and the cost of the corresponding Cycle would cost (i) + dist(i, 1) where dist(i, 1) is the distance from I to 1. Finally, we return the minimum of all [cost(i) + dist(i, 1)] values. This looks simple so far.
Now the question is how to get cost(i)? To calculate the cost(i) using Dynamic Programming, we need to have some recursive relation in terms of sub-problems.
Let us define a term C(S, i) be the cost of the minimum cost path visiting each vertex in set S exactly once, starting at 1 and ending at i . We start with all subsets of size 2 and calculate C(S, i) for all subsets where S is the subset, then we calculate C(S, i) for all subsets S of size 3 and so on. Note that 1 must be present in every subset.
Below is the dynamic programming solution for the problem using top down recursive+memoized approach:-
For maintaining the subsets we can use the bitmasks to represent the remaining nodes in our subset. Since bits are faster to operate and there are only few nodes in graph, bitmasks is better to use.
For example: –
10100 represents node 2 and node 4 are left in set to be processed
010010 represents node 1 and 4 are left in subset.
NOTE:- ignore the 0th bit since our graph is 1-based
Time Complexity : O(n 2 *2 n ) where O(n* 2 n) are maximum number of unique subproblems/states and O(n) for transition (through for loop as in code) in every states.
Auxiliary Space: O(n*2 n ), where n is number of Nodes/Cities here.
For a set of size n, we consider n-2 subsets each of size n-1 such that all subsets don’t have nth in them. Using the above recurrence relation, we can write a dynamic programming-based solution. There are at most O(n*2 n ) subproblems, and each one takes linear time to solve. The total running time is therefore O(n 2 *2 n ). The time complexity is much less than O(n!) but still exponential. The space required is also exponential. So this approach is also infeasible even for a slightly higher number of vertices. We will soon be discussing approximate algorithms for the traveling salesman problem.
Next Article: Traveling Salesman Problem | Set 2
References:
http://www.lsi.upc.edu/~mjserna/docencia/algofib/P07/dynprog.pdf
http://www.cs.berkeley.edu/~vazirani/algorithms/chap6.pdf
Please Login to comment...
Similar reads.
- Dynamic Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
traveling-salesman-problem
Here are 14 public repositories matching this topic..., muyangye / traveling_salesman_solver_google_maps.
Genetic Algorithm + Google Maps API to solve the Traveling Salesman (NP-hard) Problem. Plan your travel over 100+ places with ease.
- Updated Feb 28, 2024
PTV-Group / tutorials-pickups-and-deliveries
Optimize specified transports (pickups and deliveries) and displays the optimal routes for each of your vehicles.
- Updated Apr 18, 2024
lukix / genemo-web-demo
Example of using GeneMO library for web
- Updated Jan 7, 2023
PTV-Group / tutorials-depot-based-transports
Optimize specific transports (single depot location, customer locations with pickups and deliveries) and displays the optimal routes for each of your vehicles on the map.
globalpolicy / TSP_with_GA_module
Implementation of a Genetic Algorithm module to solve the Traveling Salesman Problem
- Updated Mar 18, 2019
chriski777 / Interactive-TSP
- Updated Apr 14, 2020
Shaurya-Sarma / traveling-sales-man-problem
This project is an interactive visualization of the Traveling Salesperson Problem that attempts to find the most optimized path using a genetic algorithm.
- Updated Jun 4, 2022
jurgendl / traveling-salesman
Traveling Salesman Algorithm
- Updated Nov 29, 2023
viniciusNoleto / Urban_Mobility_Research_UFRR
Projeto de Mobilidade Urbana com simulação de trânsito e aplicação de estratégias de mobilidade urbana
- Updated Jan 30, 2023
ThunbergOlle / Traveling-Salesman-Problem
Salesman problem solved using genetic algorithms
- Updated Jan 14, 2019
reshinto / shoptimize
Find the most optimized route in the supermarket during grocery shopping by tackling the traveling salesman problem
- Updated Jul 24, 2022
mrdiamonddirt / travelingsalesman
A Visualisation and Exploration of the traveling Salesman Problem
- Updated Aug 15, 2023
jitendrabhamare / p5js_sketch
Host p5js_sketch with githubpages
- Updated Aug 6, 2020
matt493 / Traveling-Salesperson-Simulaton
Exploring Different optimizations on traveling salesperson problem.
- Updated Jun 5, 2021
Improve this page
Add a description, image, and links to the traveling-salesman-problem topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the traveling-salesman-problem topic, visit your repo's landing page and select "manage topics."
An evolution strategy with tailor-made mutation operator for colored balanced traveling salesman problem
- Published: 07 May 2024
Cite this article
- Sebanti Majumder 1 &
- Alok Singh ORCID: orcid.org/0000-0003-3585-4957 1
24 Accesses
Explore all metrics
This paper deals with an \(\mathcal{N}\mathcal{P}\) -hard problem called the colored balanced traveling salesman problem (CBTSP), which is a variation of colored traveling salesman problem (CTSP) which in turn is a variation of multiple traveling salesman problem. To effectively solve this problem, an approach based on evolution strategy is proposed where mutation operator is designed taking into consideration the characteristics of CBTSP. The results obtained through our approach have been compared with the results of the novel genetic algorithm (NGA) which is the best approach available in the literature. The results show that our approach surpasses the results reported in the literature for majority of the instances in shorter amount of time.
This is a preview of subscription content, log in via an institution to check access.
Access this article
Price includes VAT (Russian Federation)
Instant access to the full article PDF.
Rent this article via DeepDyve
Institutional subscriptions
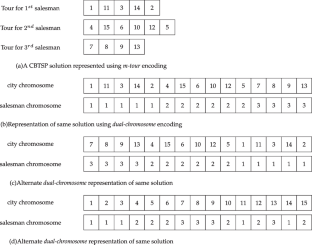
Similar content being viewed by others
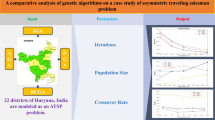
A comparative analysis of genetic algorithms on a case study of asymmetric traveling salesman problem
Hybrid Penguins Search Optimization Algorithm and Genetic Algorithm Solving Traveling Salesman Problem
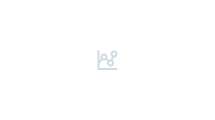
Solution to Graph Coloring Using Genetic and Tabu Search Procedures
Data availability.
All the test instances used in this paper are publicly available for download from https://leria-info.univ-angers.fr/~jinkao.hao/CTSP.html
https://mathcracker.com/wilcoxon-signed-ranks.php
Ahrari A, Kramer O (2017) Finite life span for improving the selection scheme in evolution strategies. Soft Comput 21(2):501–513
Article Google Scholar
Bäck T, Hoffmeister F, Schwefel HP (1991) A survey of evolution strategies. In: Proceedings of the fourth international conference on genetic algorithms, vol 2, pp 2–9. Morgan Kaufmann
Bartz-Beielstein T (2005) Evolution strategies and threshold selection. In: International workshop on hybrid metaheuristics, vol 3636, pp 104–115. Springer
Bektaş T (2012) Formulations and benders decomposition algorithms for multidepot salesmen problems with load balancing. Eur J Oper Res 216:83–93
Article MathSciNet Google Scholar
Bektaş T, Gouveia L, Santos D (2020) Compact formulations for multi-depot routing problems: Theoretical and computational comparisons. Comput Oper Res 12:105,084
Beyer HG, Sendhoff B (2017) Toward a steady-state analysis of an evolution strategy on a robust optimization problem with noise-induced multimodality. IEEE Trans Evol Comput 21(4):629–643
Cai J, Thierauf G (1996) Evolution strategies for solving discrete optimization problems. Adv Eng Soft 25(2–3):177–183
Carter AE, Ragsdale CT (2006) A new approach to solving the multiple traveling salesperson problem using genetic algorithms. Eur J Oper Res 175(1):246–257
Coelho VN, Coelho IM, Souza MJ, Oliveira TA, Cota LP, Haddad MN, Mladenovic N, Silva RCP, Guimarães FG (2016) Hybrid self-adaptive evolution strategies guided by neighborhood structures for combinatorial optimization problems. Evol Comput 24(4):637–666
Derrac J, García S, Molina D, Herrera F (2011) A practical tutorial on the use of nonparametric statistical tests as a methodology for comparing evolutionary and swarm intelligence algorithms. Swarm and Evol Comput 1(1):3–18
Dong W, Cai Y, Wang Y, Dong X (2016) Discrete ITÖ algorithm to the coloured travelling salesman problem. Int J Wirel Mob Comput 11(2):157–165
Dong X (2023) Hybrid ITÖ algorithm for large-scale colored traveling salesman problem. Chin J Electron 33:1–9
Google Scholar
Dong X, Cai Y (2019) A novel genetic algorithm for large scale colored balanced traveling salesman problem. Future Gener Comput Syst 95:727–742
Dong X, Dong W, Cai Y (2018) Ant colony optimisation for coloured travelling salesman problem by multi-task learning. IET Intell Trans Syst 12(8):774–782
Dong X, Lin Q, Xu M, Cai Y (2019) Artificial bee colony algorithm with generating neighbourhood solution for large scale coloured traveling salesman problem. IET Intell Trans Syst 13(10):1483–1491
He P, Hao J (2021) Iterated two-phase local search for the colored traveling salesmen problem. Eng Appl Artif Intell 97:104,018
Kashan AH, Akbari AA, Ostadi B (2015) Grouping evolution strategies: An effective approach for grouping problems. Appl Math Model 39(9):2703–2720
Li J, Dai X, Liu H, Zhou M (2015) A decomposition approach to colored traveling salesman problems. In: 2015 IEEE international conference on automation science and engineering (CASE), pp 51–56. IEEE
Li J, Meng X, Dai X (2017) Collision-free scheduling of multi-bridge machining systems: a colored traveling salesman problem-based approach. IEEE/CAA J Autom Sin 5(1):139–147
Li J, Sun Q, Zhou M, Dai X (2013) A new multiple traveling salesman problem and its genetic algorithm-based solution. In: 2013 IEEE international conference on systems, man, and cybernetics, pp 627–632. IEEE
Li J, Sun Q, Zhou M, Yu X, Dai X (2014) Colored traveling salesman problem and solution. IFAC Proc Vol 47(3):9575–9580
Li J, Zhou M, Dai X, Sun Q, Yu X (2015) A colored traveling salesman problem model for planning dual-bridge waterjet cutting paths. IEEE Transactions on Industrial Informatics
Li J, Zhou M, Sun Q, Dai X, Yu X (2014) Colored traveling salesman problem. IEEE Trans Cybern 45(11):2390–2401
Malmborg CJ (1996) A genetic algorithm for service level based vehicle scheduling. Eur J Oper Res 93(1):121–134
Pandiri V, Singh A (2018) A swarm intelligence approach for the colored traveling salesman problem. Appl Intell 48(11):4412–4428
Park YB (2001) A hybrid genetic algorithm for the vehicle scheduling problem with due times and time deadlines. Int J Prod Econ 73(2):175–188
Potvin JY (1996) Genetic algorithms for the traveling salesman problem. Ann Oper Res 63(3):337–370
Rechenberg I (1973) Evolutionsstrategie: Optimierung Technischer Systeme Nach Prinzipien der Biologischen Evolution. Frommann Holzboog Verlag, Stuttgart
Schwefel, HP (1975) Evolutionsstrategie und Numerische Optimierung. Ph.D. thesis, Technische Universität Berlin
Schwefel HP (1977) Numerische optimierung von computer-modellen mittels der evolutionsstrategie: mit einer vergleichenden einführung in die hill-climbing-und zufallsstrategie, vol 1. Springer
Singh A, Baghel AS (2009) A new grouping genetic algorithm approach to the multiple traveling salesperson problem. Soft Comput 13(1):95–101
Srivastava G, Singh A (2018) Boosting an evolution strategy with a preprocessing step: application to group scheduling problem in directional sensor networks. Appl Intell 48(12):4760–4774
Srivastava G, Singh A (2023) An evolutionary approach comprising tailor-made variation operators for rescue unit allocation and scheduling with fuzzy processing times. Eng Appl Artif Intell 123(106):246
Srivastava G, Singh A (2023) Two evolutionary approaches with objective-specific variation operators for vehicle routing problem with time windows and quality of service objectives. Appl Soft Comput 134:109,964
Srivastava G, Venkatesh P, Singh A (2020) An evolution strategy based approach for cover scheduling problem in wireless sensor networks. Int J Mach Learn Cybern 11(9):1981–2006
Tang L, Liu J, Rong A, Yang Z (2000) A multiple traveling salesman problem model for hot rolling scheduling in shanghai baoshan iron & steel complex. Eur J Oper Res 124(2):267–282
Tinós R, Helsgaun K, Whitley D (2018) Efficient recombination in the lin-kernighan-helsgaun traveling salesman heuristic. In: Parallel Problem Solving from Nature–PPSN XV: 15th International Conference, Coimbra, Portugal, September 8–12, 2018, Proceedings, Part I 15, pp 95–107. Springer
Tinós R, Whitley D, Ochoa G (2020) A new generalized partition crossover for the traveling salesman problem: tunneling between local optima. Evol Comput 28(2):255–288
Wang Y, Dong W, Dong X (2018) A novel ITÖ algorithm for influence maximization in the large-scale social networks. Future Gener Comput Syst 88:755–763
Whitley D, Hains D, Howe A (2010) A hybrid genetic algorithm for the traveling salesman problem using generalized partition crossover. In: Parallel problem solving from nature, PPSN XI: 11th International Conference, Kraków, Poland, September 11-15, 2010, Proceedings, Part I 11, pp 566–575. Springer
Wierstra D, Schaul T, Glasmachers T, Sun Y, Peters J, Schmidhuber J (2014) Natural evolution strategies. J Mach Learn Res 15(1):949–980
Wilcoxon F, Katti S, Wilcox RA (1970) Critical values and probability levels for the wilcoxon rank sum test and the wilcoxon signed rank test. Inst Math Stat Collect 1:171–259
Zhang P, Wang J, Tian Z, Sun S, Li J, Yang J (2022) A genetic algorithm with jumping gene and heuristic operators for traveling salesman problem. Appl Soft Comput 127:109,339
Download references
Author information
Authors and affiliations.
School of Computer and Information Sciences, University of Hyderabad, Hyderabad, 500 046, Telangana, India
Sebanti Majumder & Alok Singh
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Alok Singh .
Ethics declarations
Conflicts of interest.
The authors declare that they have no conflict of interest.
Ethical approval
This article does not contain any studies with human participants or animals performed by any of the authors.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Rights and permissions
Springer Nature or its licensor (e.g. a society or other partner) holds exclusive rights to this article under a publishing agreement with the author(s) or other rightsholder(s); author self-archiving of the accepted manuscript version of this article is solely governed by the terms of such publishing agreement and applicable law.
Reprints and permissions
About this article
Majumder, S., Singh, A. An evolution strategy with tailor-made mutation operator for colored balanced traveling salesman problem. Appl Intell (2024). https://doi.org/10.1007/s10489-024-05473-3
Download citation
Accepted : 19 April 2024
Published : 07 May 2024
DOI : https://doi.org/10.1007/s10489-024-05473-3
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Colored balanced traveling salesman problem
- Colored traveling salesman problem
- Evolution strategy
- Traveling salesman problem
- Find a journal
- Publish with us
- Track your research

IMAGES
VIDEO
COMMENTS
Challenge in Detail. Create a function that solves the Traveling Salesman Problem using a genetic algorithm. The function should take a list of cities and their coordinates as input and return the shortest possible route, visiting each city exactly once and returning to the starting city. The genetic algorithm should involve selection ...
The Traveling Salesman Problem states that you are a salesperson and you must visit a number of cities or towns. The Traveling Salesman Problem. Rules: Visit every city only once, then return back to the city you started in. Goal: Find the shortest possible route. Except for the Held-Karp algorithm (which is quite advanced and time consuming ...
Node.js is built on the V8 JavaScript engine from Google Chrome and provides an event-driven, non-blocking I/O model that makes it lightweight and efficient. Travelling Salesman Problem (TSP) The Travelling Salesman Problem (TSP) is a classic algorithmic problem in the fields of computer science and operations research. It focuses on ...
Travelling Salesman Problem (TSP) : Given a set of cities and distances between every pair of cities, the problem is to find the shortest possible route that visits every city exactly once and returns to the starting point. Note the difference between Hamiltonian Cycle and TSP. The Hamiltonian cycle problem is to find if there exists a tour that visits every city exactly once.
Pull requests. This project uses a Genetic Algorithm to find near-optimal solutions to the Traveling Salesman Problem (TSP). Users can visualize the evolving routes and compare them to the optimal solution found using Bruteforce. visualization javascript genetic-algorithm travelling-salesman-problem. Updated on Oct 25, 2023.
The Travelling Salesman Problem also known as TSP is an NP-hard problem in combinatorial optimization. Imagine a set of city disposed on a map, you have a set of salesman (population) and they must all go to every city in the least amount of time/distance.
The travelling salesman problem (TSP) asks the following question: Given a list of cities and the distances between each pair of cities, what is the sh... Pen Settings. ... JavaScript preprocessors can help make authoring JavaScript easier and more convenient. Learn more · Versions
Traveling Salesperson Problem. This section presents an example that shows how to solve the Traveling Salesperson Problem (TSP) for the locations shown on the map below. The following sections present programs in Python, C++, Java, and C# that solve the TSP using OR-Tools.
The function takes two Point objects as arguments and returns a number for distance. Defaults to simple Euclidean distance calculation. Example. var points = [ new salesman.Point(2,3) //other points ]; var solution = salesman.solve(points); var ordered_points = solution.map(i => points[i]); // ordered_points now contains the points, in the ...
Traveling Salesman Problem. This is a TSP solver in javascript that uses d3.js for visualization. Click a bunch of spots on the map to make "cities", then click "Run" to run the TSP solver. It will display its first guess, then its final guess. See if it does well. (Note, it minimizes flat x/y Euclidean distances, America is there for effect ...
The traveling salesman problem (TSP) is a widely studied combinatorial optimization problem, which, given a set of cities and a cost to travel from one city to another, seeks to identify the tour that will allow a salesman to visit each city only once, starting and ending in the same city, at the minimum cost. 1.
TLDR version: Most important issue is, that in a TSP problem, instead of finding the shortest Hamiltonian cycle, what are good ways to find the best path (I suppose the one that visits the most nodes) which is at most X length, with a fixed starting point. Full version: I'm interested in some ideas for a problem that involves TSP. First, an example real-world TSP problem is when you have N ...
This blog helps in understanding underlying javascript concepts, problems, competitive programming and javascript related frameworks. travelling salesman problem in javascript Home
traveling-salesman-problem. A solution with Javascript to a typical Traveling Salesman Problem using bit operations and Dynamic Programming. Description. This is a famous problem of finding the shortest path when visiting multiple nodes only once. The distance between nodes (movement cost between nodes) is given as a condition. Cost definition
Travelling Salesman Problem (TSP): Given a set of cities and distance between every pair of cities, the problem is to find the shortest possible route that visits every city exactly once and returns back to the starting point. Note the difference between Hamiltonian Cycle and TSP. The Hamiltonian cycle problem is to find if there exist a tour that visits every city exactly once.
Travelling Salesman Problem with Code. Given a set of cities(nodes), find a minimum weight Hamiltonian Cycle/Tour. Concepts Used:. Graphs, Bitmasking, Dynamic Programming
Approach: This problem can be solved using Greedy Technique. Below are the steps: Create two primary data holders: A list that holds the indices of the cities in terms of the input matrix of distances between cities. Result array which will have all cities that can be displayed out to the console in any manner.
The Traveling Salesman Problem (TSP) is a problem of determining the most efficient route for a round trip, with the objective of maintaining the minimum cost and distance traveled. It serves as a foundational problem to test the limits of efficient computation in theoretical computer science. The salesman's objective in the TSP is to find a ...
Travelling Salesman Solver A TSP Solver using D3.js that generates random points and implements various heuristics to find a solution. Heuristics include hill climber, simulated annealing, two-opt edge swap, nearest neighbor, nearest insertion, and farthest insertion.
Travelling Salesman Problem in JavaScript Canvas. Posted by joe | Filed under Javascript, Software Development. A long a time ago - when I was student at university - I wrote a small, but somewhat impressing program. It is a heuristic (and thus suboptimal) solver for the travelling salesman problem, using an algorithm from the field of ...
The problem is a famous NP-hard problem. There is no polynomial-time know solution for this problem. The following are different solutions for the traveling salesman problem. Naive Solution: 1) Consider city 1 as the starting and ending point. 2) Generate all (n-1)!
Language: JavaScript. Filter by language. ... Find the most optimized route in the supermarket during grocery shopping by tackling the traveling salesman problem. react redux material-ui traveling-salesman-problem Updated Jul 24, 2022; JavaScript; viniciusNoleto / Urban_Mobility_Research_UFRR Star 0. Code ...
This paper deals with an \(\mathcal{N}\mathcal{P}\)-hard problem called the colored balanced traveling salesman problem (CBTSP), which is a variation of colored traveling salesman problem (CTSP) which in turn is a variation of multiple traveling salesman problem.To effectively solve this problem, an approach based on evolution strategy is proposed where mutation operator is designed taking ...